What is SystemJS?
SystemJS is a Javascript library that transforms the browser into a DOS terminal. The library provides an interface to the developer to print any printable ASCII character and choose the color and background color for them.
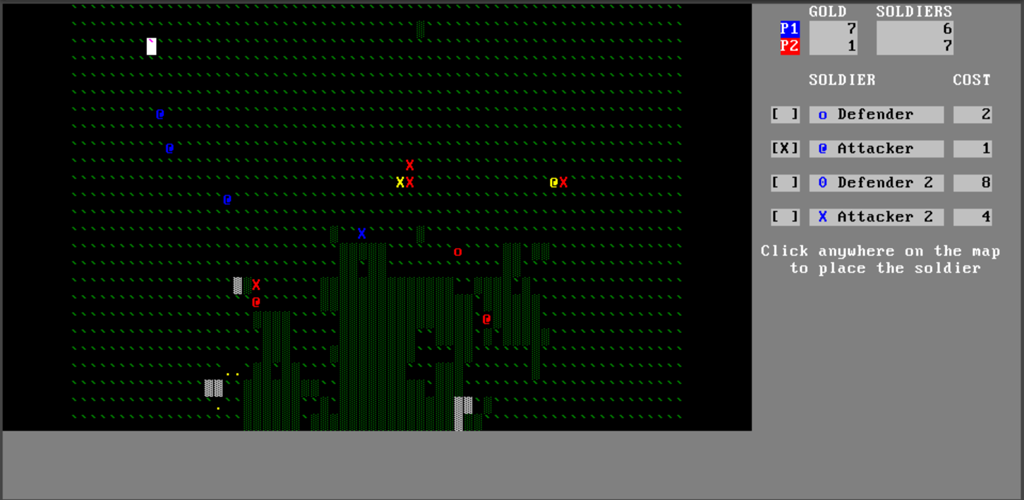
Getting Started
Setting up SystemJS is very simple, just include the JS file and the CSS file and your browser will automatically show the DOS screen on the page.
Add the CSS file to your document's head:
<link href="path/to/systemjs/system.css" rel="stylesheet">
Add the JS file anywhere in the document:
<script src="path/to/systemjs/system.js"></script>
If you view the page in a browser now, you should see a black screen with a little white rectangle indicating where the mouse is.
Using SystemJS
Now that you've set it up, add your own scripts anywhere after the SystemJS file has been included.
Every property and method available to you is in the global system object.
Properties
- system.width and system.height
This indicates how wide and tall the viewport is in characters. If the width is 64, that means you can put up to 64 letters on the row before it wraps to the next line.
- system.mouseX and system.mouseY
The indicates the coordinates of the mouse cursor on the viewport measured in characters.
Methods
- system.cls()
Resets the entire screen, setting the background of every grid cell black, the color white and erasing any printed characters.
- system.color(int text [, int background])
-
Sets the color of the text and, optionally, of the background for anything printed after this command is executed. If the background is not specified, the previously selected background color will be used. By default text is white and the background is black.
The text and background parameters take the following color codes:
Code Hex Color Code Hex Color 0 0x0 Black 8 0x8 Dark Grey 1 0x1 Blue 9 0x9 Light Blue 2 0x2 Green 10 0xA Lime 3 0x3 Teal 11 0xB Cyan 4 0x4 Maroon 12 0xC Red 5 0x5 Purple 13 0xD Magenta 6 0x6 Olive 14 0xE Yellow 7 0x7 Light Grey 15 0xF White - system.locate(int row, int column)
-
Moves the cursor for the next piece of text output to the specified row and column. The order of parameters is row, then column, which in cartesian would be Y and then X. Keep this is mind when using the locate() method.
If this command has never been used, the cursor will be one cell after the last character that was printed.
- system.print([string text])
-
Prints text at the current cursor position. The cursor starts at (0, 0) when the system starts up but may be moved by system.locate() and system.print() commands.
If this method is called with no parameters it will move the cursor to the beginning of the next row.
This method has access to all the original ASCII extended characters, including box pieces and dotted rectangles. Some of these are printed differently in Unicode, so here is a list of escape codes for all the extended characters. Copy and paste the code into your strings in Javascript to print these characters.
Char Code Char Code Char Code Char Code Char Code Char Code \xA0 ° \xB0 À \xC0 Ð \xD0 à \xE0 ð \xF0 ¡ \xA1 ± \xB1 Á \xC1 Ñ \xD1 á \xE1 ñ \xF1 ¢ \xA2 ² \xB2 Â \xC2 Ò \xD2 â \xE2 ò \xF2 £ \xA3 ³ \xB3 Ã \xC3 Ó \xD3 ã \xE3 ó \xF3 ¤ \xA4 ´ \xB4 Ä \xC4 Ô \xD4 ä \xE4 ô \xF4 ¥ \xA5 µ \xB5 Å \xC5 Õ \xD5 å \xE5 õ \xF5 ¦ \xA6 ¶ \xB6 Æ \xC6 Ö \xD6 æ \xE6 ö \xF6 § \xA7 · \xB7 Ç \xC7 × \xD7 ç \xE7 ÷ \xF7 ¨ \xA8 ¸ \xB8 È \xC8 Ø \xD8 è \xE8 ø \xF8 © \xA9 ¹ \xB9 É \xC9 Ù \xD9 é \xE9 ù \xF9 ª \xAA º \xBA Ê \xCA Ú \xDA ê \xEA ú \xFA « \xAB » \xBB Ë \xCB Û \xDB ë \xEB û \xFB ¬ \xAC ¼ \xBC Ì \xCC Ü \xDC ì \xEC ü \xFC \xAD ½ \xBD Í \xCD Ý \xDD í \xED ý \xFD ® \xAE ¾ \xBE Î \xCE Þ \xDE î \xEE þ \xFE ¯ \xAF ¿ \xBF Ï \xCF ß \xDF ï \xEF ÿ \xFF - system.wait(function callback, int ms)
This is an alias for Javascript's setTimeout() method.
-
system.keydown(function callback [, bool remove])
system.keyup(function callback [, bool remove]) -
This method assigns or removes a keydown or keyup event listener.
callback is a function with one parameter, this parameter holds the key code for the key that was pressed (keydown method) or released (keyup method) to fire the event.
If the remove parameter is omitted or set to false, when a key is pressed (keydown method) or released (keyup method) the function given in callback will be called. If the removeparameter is set to true then the event listener will be removed.
-
system.mousedown(function callback [, bool remove])
system.mouseup(function callback [, bool remove]) -
This method assigns or removes a mousedown or mouseup event listener.
callback has no parameters for the mouse events, instead you can access the global system.mouseX and system.mouseY properties to determine where the mouse was when the event occurred.
If the remove parameter is omitted or set to false, when a mouse button is pressed (mousedown method) or released (mouseup method) the function given in callback will be called. If the removeparameter is set to true then the event listener will be removed.